This article will demonstrate how to get you started with
KickApps REST API methods. This tutorial covers requesting and
basic use of the token. Hopefully you can use this project as base
for all your KickApps data integration, since most of the calls are
pretty much the same, the only change is the url of a particular
method and post parameters you are passing to it.
Having recently done quite an interesting project for a client
(auto import of news items from kickapps to umbraco including media
items download/reupload to umbraco with media files paths auto
replaced) I realised that isn't much struff online on KickApps/C#
topic.
Visual Studio Setup
First of all, lets create a new project in VS 2010. Make
sure the project runs on .NET 4. In your visual studio
2010 click File -> New Project and select ASP.NET Empty Web
Application. In the project name field enter KickAppsEmptySample.
Once you've got your solution and project initialised, in Solution
Explorer window (View -> Solution Explorer), right click on the
project and select Add -> New Folder (we need 2 folders -
Model and Provider).
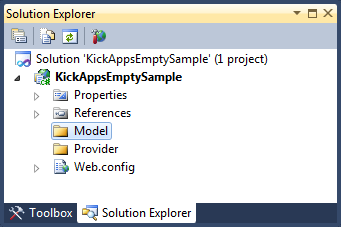
We are going to use our Model folder as a base for all our
classes to which we convert the JSON response from KickApps. See http://www.kickapps.com/documentation/index.php/Create_Token
for more details.
Now, we're going to store the login credentials in the
web.config, make sure your web.config contains following keys:
<?xml version="1.0"?>
<configuration>
<system.web>
<compilation debug="true" targetFramework="4.0" />
</system.web>
<appSettings>
<add key="KickAppsSiteId" value="XXX" />
<add key="KickAppsUser" value="YYY" />
<add key="DeveloperKey" value="ZZZ" />
</appSettings>
</configuration>
KickAppsUser is either your email address or
username.
Model
Now create following classes in our Model folder (Add -> New
Item -> Class):
Token.cs
namespace KickAppsEmptySample.Model
{
public class Token
{
public string TOKEN;
public string METHOD;
public string PRIVILEGES;
public string payload_type;
public int userId;
public string email;
public string username;
}
}
Result.cs
namespace KickAppsEmptySample.Model
{
public class Result
{
public string errorMessage;
public ReturnCode returnCode;
public enum ReturnCode
{
Unknown = 0,
Success = 1,
Failure
}
}
}
Each API call returns a different JSON object (sometimes eg.
search, may return an object that contains an array of other
objects), you need a C# class for each type of the object your API
call will return. Result.cs is only there for a
standarised way of returning a method call result - you will see it
in action in a sec.
Provider
Now, let's create our KickApps (KickApps.cs)
provider under Provider folder.
using System;
using System.Collections.Generic;
using System.IO;
using System.Configuration
using System.Net;
using System.Text;
using KickAppsEmptySample.Model;
namespace KickAppsEmptySample.Provider
{
public class KickApps
{
#region URLS FOR API CALLS
private const string CreateTokenUrl = "http://api.kickapps.com/rest/token/create/";
#endregion
/// <summary>
/// The site that you are searching. Each affiliate site has a unique as.
/// </summary>
private string siteId;
/// <summary>
/// email address or username - required for token request
/// </summary>
private string user;
/// <summary>
/// developer key - required for token request
/// </summary>
private string developerKey;
/// <summary>
/// Token object - contains unique TOKEN property generated by KickApps
/// </summary>
public Token token;
/// <summary>
/// set siteId, user (password or username) and developerKey on object initialisation
/// </summary>
public KickApps()
{
this.siteId = ConfigurationManager.AppSettings["KickAppsSiteId"];
this.user = ConfigurationManager.AppSettings["KickAppsUser"];
this.developerKey = ConfigurationManager.AppSettings["DeveloperKey"];
}
}
}
Before we start making the actual API calls, we need to create 2
methods that are quite handy and will be used for every API
call.
BuildPostString will build a post string based
on the dictionary items you pass to it (key = value).
private string BuildPostString(Dictionary<string, string> postValues)
{
StringBuilder sb = new StringBuilder();
StringBuilder sbDebug = new StringBuilder();
foreach (KeyValuePair<string, string> value in postValues)
{
if (sb.Length > 0) sb.Append("&");
sb.Append(string.Format("{0}={1}", value.Key, value.Value));
}
return sb.ToString();
}
SubmitPostRequest will submit the request
to the specified url.
private Result SubmitPost(string url, string postValues, out string response)
{
Result result = new Result ();
response = string.Empty;
try
{
ASCIIEncoding encoding = new ASCIIEncoding();
byte[] data = encoding.GetBytes(postValues);
HttpWebRequest webRequest = (HttpWebRequest)WebRequest.Create(url);
webRequest.Method = "POST";
webRequest.ContentType = "application/x-www-form-urlencoded";
webRequest.Timeout = System.Threading.Timeout.Infinite;
webRequest.ContentLength = data.Length;
Stream requestStream = webRequest.GetRequestStream();
requestStream.Write(data, 0, data.Length);
requestStream.Close();
requestStream.Flush();
WebResponse webResponse = webRequest.GetResponse();
StreamReader reader = new StreamReader(webResponse.GetResponseStream());
var dataReceived = reader.ReadToEnd();
webResponse.Close();
reader.Close();
webRequest.Abort();
result.returnCode = Result.ReturnCode.Success;
response = dataReceived;
}
catch (Exception ex)
{
result.returnCode = Result.ReturnCode.Failure;
result.errorMessage = ex.Message;
}
return result;
}
Once we've got both methods created, we can acually make out
first REST API call. CreateToken() will request the token needed
for all our API calls and save it in the Token object. I've tried
to put as many comments as possible so you know what each bit
does.
/// <summary>
/// creates a token object (which contains TOKEN property erquired by all API calls
/// </summary>
public void CreateToken()
{
// builds the final url that post values will be sent to,
// if you had a look at the REST documentation on kickapps.com,
// you will notice that create token url format is
// http://api.kickapps.com/rest/token/create/{username}/{as} or http://api.kickapps.com/rest/token/create/{email}/{as}
StringBuilder url = new StringBuilder();
url.Append(CreateTokenUrl);
url.Append(user);
url.Append(string.Format("/{0}", siteId));
// now specify all the post parameters you want to sent to the url you created above
// according to the API documentation (http://www.kickapps.com/documentation/index.php/Create_Token)
// CreateToken API call requires developerKey, and 3 other optional parameters
Dictionary<string, string> postValues = new Dictionary<string, string>();
postValues.Add("developerKey", developerKey);
// response will contain a JSON response
string response;
var header = SubmitPostRequest(url.ToString(), BuildPostString(postValues), out response);
if (header.returnCode == Result.ReturnCode.Success)
{
// convert JSON response to our Model/Token oject
token = (Token)Newtonsoft.Json.JsonConvert.DeserializeObject(response, typeof(Token));
}
}
If you build this project, it will complain about missing .dll -
it's Newtonsoft.Json.dll. I've attached the dll to
this article so simply unzip it and drop into the /bin directory
and add as a reference to your project.
Now to test this project, add a web form to it
Default.aspx and paste following code into it:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="KickAppsEmptySample.Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="btnGenerateToken" runat="server" Text="Generate Token"
onclick="btnGenerateToken_Click" />
<asp:Literal ID="litResult" runat="server"></asp:Literal>
</div>
</form>
</body>
</html>
and Default.aspx.cs
namespace KickAppsEmptySample
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnGenerateToken_Click(object sender, EventArgs e)
{
KickAppsEmptySample.Provider.KickApps ka = new Provider.KickApps();
ka.CreateToken();
if (ka.token != null && ka.token.TOKEN != string.Empty) litResult.Text = ka.token.TOKEN;
}
}
}
Once you've got your token, you can call any API method
supplying correct querystring and post parameters in idential way
as create token method described in this article. I'd suggest to
inspect the output (JSON) for object properties when you create
your C# class to which you convert JSON response.
Tagged: .NET 4
C#
JSON
kickapps api
REST
web programming
windows development